Java 8 Stream - Sort HashMap based on Keys and Values
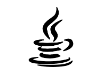
By default, Java HashMap doesn’t maintain any order. However, if you need to sort the HashMap, we sort the HashMap explicitly based on your requirements. So, in this section, let’s understand how to sort the hashmap according to the keys and values by using Java 8’s Stream API. 1. Sort HashMap by keys in natural order import java.util. *; import java.util.stream.Collectors ; public class Main { public static void main ( String [] args) { Map < Integer , String > map = new HashMap<>(); map .put( 44 , "Java" ); map .put( 66 , "Ada" ); map .put( 29 , "Go" ); map .put( 98 , "Kotlin" ); map .put( 11 , "C" ); map .put( 125 , "Rust" ); Map < Integer , String > sortedMap = map .entrySet() .stream() .sorted( Map . Entry . comparingByKey ()) .collect( Collectors . toMap ( Map . Entry ::get