Java 8 Streams - Count Frequency Of Words In a List
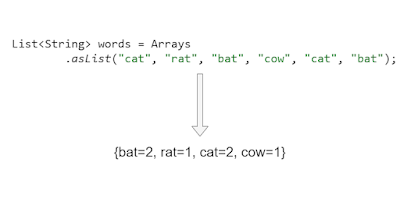
Example 1 import java.util.Arrays ; import java.util.List ; import java.util.Map ; import java.util.function.Function ; import java.util.stream.Collectors ; public class Main { public static void main ( String [] args) { List < String > words = Arrays . asList ( "cat" , "rat" , "bat" , "cow" , "cat" , "bat" ); // For Long values Map < String , Long > result = words .stream() .collect( Collectors . groupingBy ( Function . identity (), Collectors . counting ())); System . out .println( result ); } } The List interface in Java provides a way to store the ordered collection. It is a child interface of Collection. It is an ordered collection of objects in which duplicate values can be stored. The asList () method of java.util.Arrays class is used to return a fixed-size list backed by the specified array. This metho