Spring Boot + Angular: File Upload & Download Example
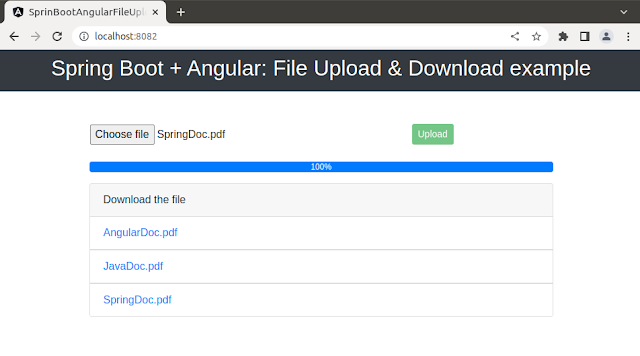
Hello everyone, today we will learn how to upload and download file with Spring Boot and Angular. You can download the source code from our GitHub repository. Backend Project Directory: Frontend Project Directory: We will build two projects: 1. Backend: springboot-fileupload-filedownload 2. Frontend: angular-file-upload Project 1: springboot-file-upload-download pom.xml <?xml version = "1.0" encoding = "UTF-8" ?> <project xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns = "http://maven.apache.org/POM/4.0.0" xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd" > <modelVersion> 4.0.0 </modelVersion> <parent> <groupId> org.springframework.boot </groupId> <artifactId> spring-boot-starter-parent </artifactId> <version> 2.7.0 </version> <!-- lookup parent fro