Spring Boot - Spring Security - Okta OAuth2 Login - Example
Hello everyone, Today we are going to learn how to integrate the Okta OAuth2 Sign-In by utilizing the Spring Boot application.
More Spring Security topics:
Generate Okta OAuth2 credentials
1. Register Okta developer account - click here
2. Customize your goals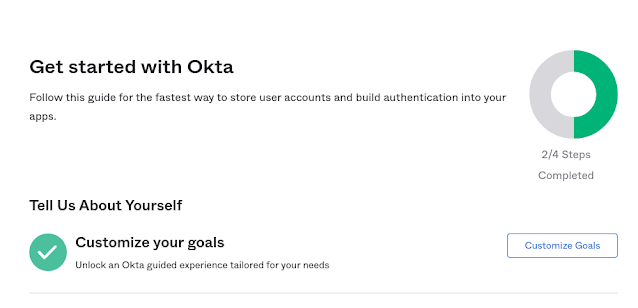
3. After clicking the Customize Goals, one modal will pop up "Tell Us About Yourself"
6. After clicking the Create New App, one modal will pop up "Create a new app integration"
8. After clicking the Save button, System will generate the Client ID and Client Secret and Okta domain.
Creating a Simple Web Application
Now we are going to develop a simple web application using Spring Security and Okta.
Project Structure:
Project Dependency(pom.xml):
A Project Object Model or POM is the fundamental unit of work in Maven. It is an XML file that contains information about the project and configuration details utilized by Maven to build the project. It contains default values for most projects. Some of the configurations that can be designated in the POM is the project dependencies, the plugins or goals that can be executed, the build profiles, and so on. Other information such as the project version, description, developers, mailing lists, and such can withal be designated.
<?xml version="1.0" encoding="UTF-8"?><project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.5.5</version> <relativePath /> <!-- lookup parent from repository --> </parent> <groupId>com.knf.dev.demo</groupId> <artifactId>springOauth2Okta</artifactId> <version>0.0.1-SNAPSHOT</version> <name>springOauth2Okta</name> <description>Demo project for Spring Boot</description> <properties> <java.version>11</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.thymeleaf.extras</groupId> <artifactId>thymeleaf-extras-springsecurity5</artifactId> </dependency> <dependency> <groupId>com.okta.spring</groupId> <artifactId>okta-spring-boot-starter</artifactId> <version>2.1.1</version> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-test</artifactId> <scope>test</scope> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build></project>
Enable Okta Sign-in (Spring Magic):
okta: oauth2: issuer: https://<domain>/oauth2/default client-secret: <client-secret> client-id: <client-id>spring: thymeleaf: cache: 'false'
WebController:
package com.knf.dev.demo.controller;
import java.security.Principal;import org.springframework.stereotype.Controller;import org.springframework.web.bind.annotation.GetMapping;
@Controllerpublic class WebController {
@GetMapping("/") public String index(Principal principal) { return "index"; }}
Main class:
package com.knf.dev.demo;
import org.springframework.boot.SpringApplication;import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplicationpublic class SpringOauth2OktaApplication {
public static void main(String[] args) { SpringApplication.run(SpringOauth2OktaApplication.class, args); }}
The @SpringBootApplication annotation is a convenience annotation that combines the @EnableAutoConfiguration, @Configuration and the @ComponentScan annotations.
View(index.html)
<!DOCTYPE html><html xmlns="http://www.w3.org/1999/xhtml" xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3" xmlns:th="https://www.thymeleaf.org"><head> <title>Spring Boot OAuth2 Login with Okta - Demo</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script><script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"> </script></head><body><nav class="navbar navbar-inverse"> <div class="container-fluid"> <div class="navbar-header"> <button class="navbar-toggle" data-target="#myNavbar" data-toggle="collapse" type="button"> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand" href="#">Logo</a> </div> <div class="collapse navbar-collapse" id="myNavbar"> <ul class="nav navbar-nav"> <li class="active"><a href="#">Home</a></li> </ul> </div> </div></nav>
<div class="container-fluid text-center"> <div class="row content"> <div class="col-sm-2 sidenav"> </div> <div class="col-sm-8 text-left"><h1>Welcome <span th:text="${#authentication.principal.attributes.name}"> </span> </h1> <p>You have been successfully logged in</p> </div> </div></div></body></html>
Run & Test
Github repository download link is provided at the end of this tutorial
Github repository download link is provided at the end of this tutorial
Local Setup:
Step 1: Download or clone the source code to a local machine.
Step 2: mvn clean install
Step 3: Run the Spring Boot applicationmvn spring-boot:run
mvn spring-boot:run
Access the URL in the browser: http://localhost:8080/
The application will redirect the user to the Okta login screen